.Js
Document Object Model
The JavaScript Document Object Model (DOM) is a programming interface for web documents. It represents the structure of HTML and XML documents as a tree-like structure, where each node represents a part of the document, such as an element, attribute, or text.
Overview of working with the DOM in JavaScript:
Accessing Elements:
You can access elements in the DOM using methods like 'document.getElementById', 'document.getElementsByClassName', 'document.getElementsByTagName, or more modern methods like 'document.querySelector' and 'document.querySelectorAll'.
Manipulating Elements:
Once you have a reference to an element, you can manipulate it by changing its properties, attributes, or content. For example, you can change the 'textContent', 'innerHTML', 'style', or 'className' properties.
Creating and Appending Elements:
You can create new elements using the 'document.createElement' method and append them to the document using methods like 'appendChild', 'insertBefore', or 'insertAdjacentHTML'.
Event Handling:
You can attach event listeners to elements to respond to user interactions. Common events include click, mouseover, keydown, etc. You can use the 'addEventListener' method to register event handlers.
Traversal and Navigation:
You can navigate through the DOM tree using properties like 'parentNode', 'childNodes', 'firstChild', 'lastChild', 'nextSibling', and 'previousSibling'.
CSS Selectors:
With modern methods like 'querySelector' and 'querySelectorAll', you can use CSS selectors to easily select elements in the DOM.
Demonstrating some of these concepts:
This example demonstrates some basic DOM manipulation techniques in JavaScript.
<!DOCTYPE html> <html> <head> <title>DOM Example</title> <style> body{ background: #333; } </style> </head> <body> <div id="container"> <h1>Hello, DOM!</h1> <p>This is a paragraph.</p> </div> <script> // Accessing an element by id var container = document.getElementById('container'); // Modifying styles container.style.color = '#fff'; container.style.fontSize = '20px'; // Manipulating content container.innerHTML += '<p>This is another paragraph.</p>'; // Creating new element var newDiv = document.createElement('div'); newDiv.textContent = 'This is a new div'; container.appendChild(newDiv); // Event handling newDiv.addEventListener('click', function() { alert('New div clicked!'); }); </script> </body> </html>
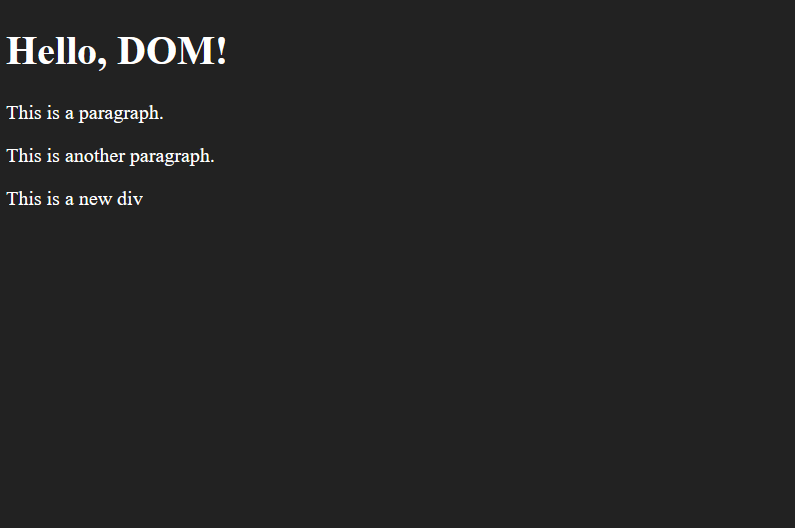
What's Next?
We actively create content for our YouTube channel and consistently upload or share knowledge on the web platform.